Strange Depths
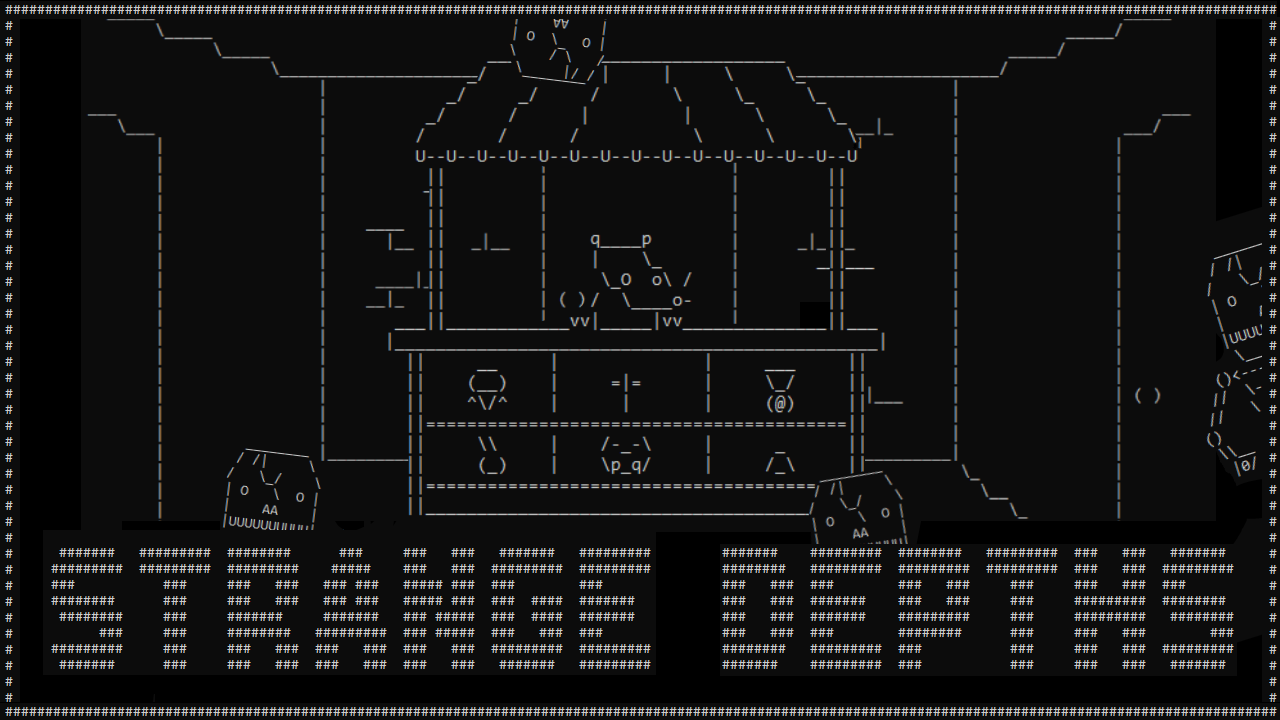
My Responsibilities
Rendering Systems
Animation Systems
Player Input Systems
Gameplay Systems
Tools
SourceTree
Visual Studio
Git
Game Overview
Strange Depths is a 2D turn-based Dungeon Crawler all rendered through the Windows terminal. You must escape the Non-Eucledian maze you've trapped yourself in by collecting enough coins to buy the Keystone while surviving endless waves of enemies.
Experience Summary
Strange Depths is a portfolio project done to demo my skills and knowledge of C++. This was a solo project and I was responsible to creating/designing all the technical and gameplay features in the game. I was also the artist and created all the characters and animations. Working alone meant that I had to be more organized and self motivated as I did not have team members to rely on this time.
This project has taught me a lot about how to use C++ efficiently and better coding practices, so I could add more features much easier in the future. Programming this game was a struggle as this was the first C++ project I have ever created and wanting to create low level features was not a good mix. But, through my determination, excellent problem solving and researching skills I managed to pull through and complete the game.
Rendering Systems
Creating a rendering system was no easy task and took many iterations to get to where it is currently. I took some inspiration from existing game engines, such as Unity, to create my own system. Firstly, I made a seperate rendering thread, so the gameplay would not get hung up on the rendering and vice versa. Through testing I found that printing on the the screen was quite slow and expensive, so to counteract that I created a "buffer frame" system. The "buffer frame" system calculates what each character should be in each spot on the console, then once it's done it prints it all onto the screen at once. I really wanted my images to have transparency, so in order to do that I made my system render the images through a priority system, allowing for images to be rendered above each other sequentially as well as allowing for transparency. Lastly, I wanted it to be easy for me to add/remove/change images, so I made the images I want to be rendered pointers, which allows me to do all that without having to worry about touching the render thread.
Animation Systems
To create my animation system, I took some inspirations from Unity's Animator system. To better organize my thinking I created a task list, which consisted of: Animator, Animation Clip, Transistions, and Conditions. I tackled the animation clips first, and created a sequence of images which would act as keyframes and would be used when the frame was reached in the Animator. Then I created the animator which is just a container for all the animation system's objects as well as a loop which would loop through it's current animation on another thread. However, putting the animation loop on a seperate thread caused issues for when the animator would be deleted as it would loose reference, so I made the destructor wait for the loop to finish before destroying the animator. Lastly, I created transistions and conditions which would be check for the correct conditions in the Animator and if the condition was met it would transition to the desired clip.
Player Input Systems
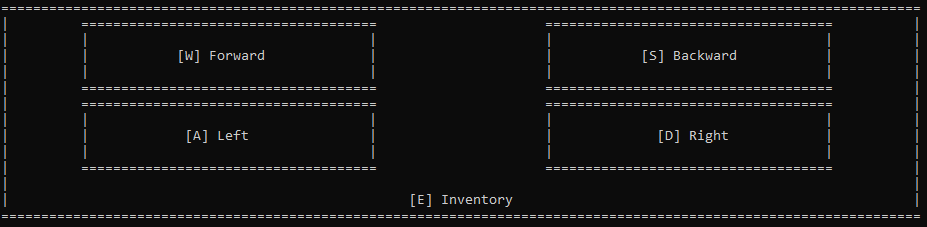
I really struggled with coming up with a design for an input system where it would facilitate different actions with the input of the same keys when in different menus. After doing some research on data structures I could use, I came across the Stack structure and it fit perfectly into the sort of system I wanted.
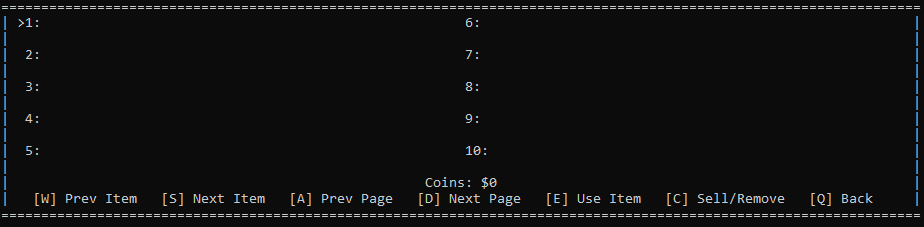
The avaible player actions would be stored in an action map and when said action map would be needed I would be able to add it to the stack and when finished I would pop it off and it would resume the previous action map. This allowed for a large simplification in my code as I was able to call all my actions through one main action loop.
Gameplay Systems
Gameplay systems were surprisingly hard to code as I wasn't used to the header file system which forced me to code in a much more decoupled manner than I have had to before. For my game I wanted to have chests and enemies drop loot, so to avoid having to code drop mechanics in each enemy and chests I created a loot table system which handles the types of loot as well as how the loot generates. I also had to create an Event System in the game to help facilitate my buff system which I managed to solve from researching and understanding the Observer Coding Pattern.